1. Introduction#
1.1. Scientific Computing#
https://en.wikiversity.org/wiki/Scientific_computing https://www.nature.com/articles/d41586-024-00725-1
1.2. High Performance Scientific Computing#
https://en.wikipedia.org/wiki/High-performance_computing?useskin=vector

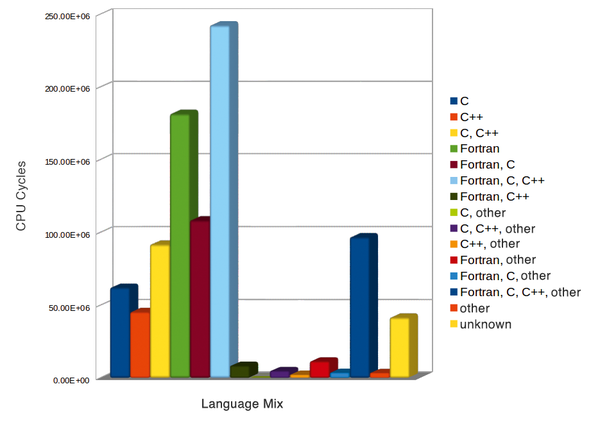
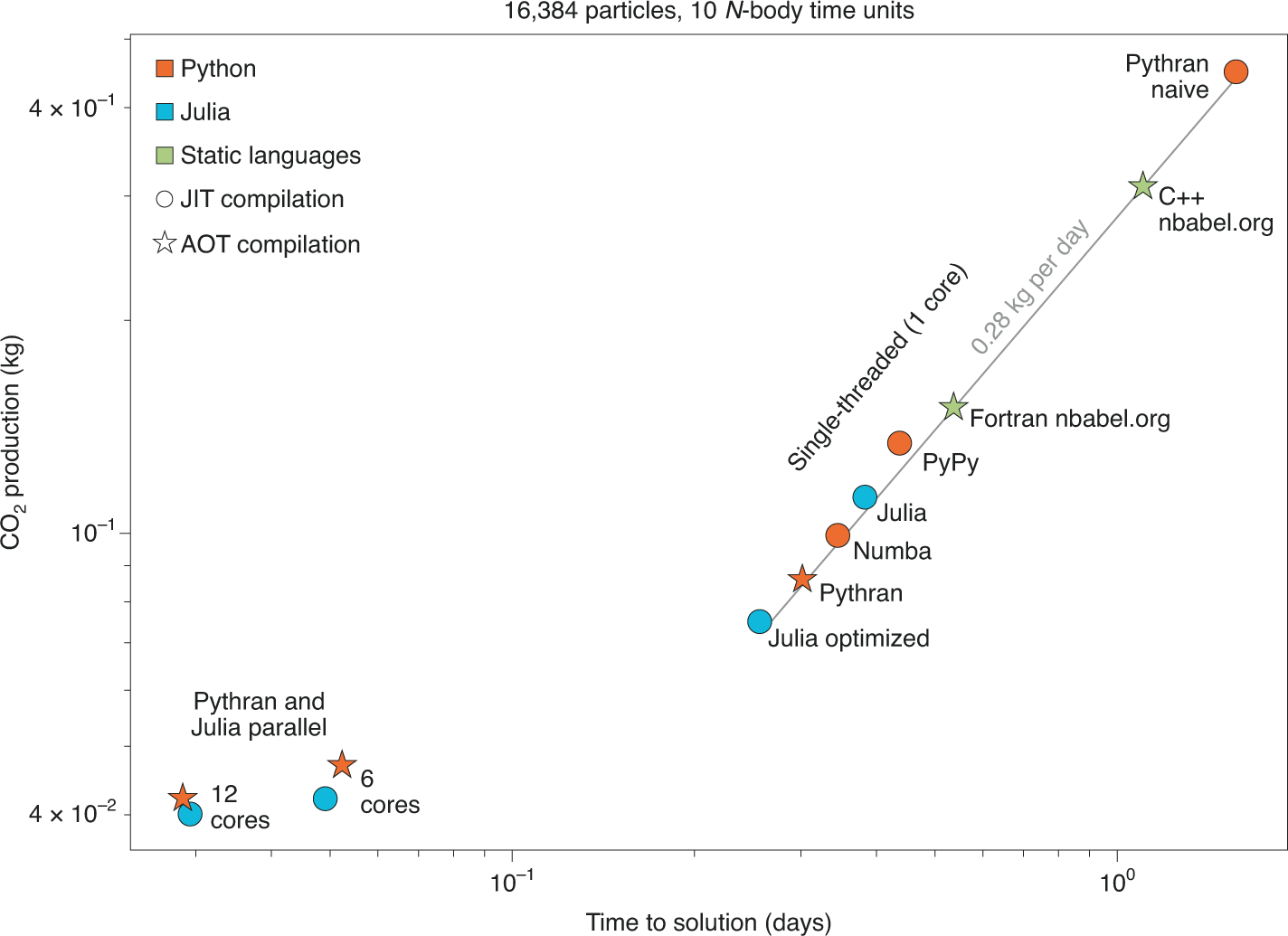
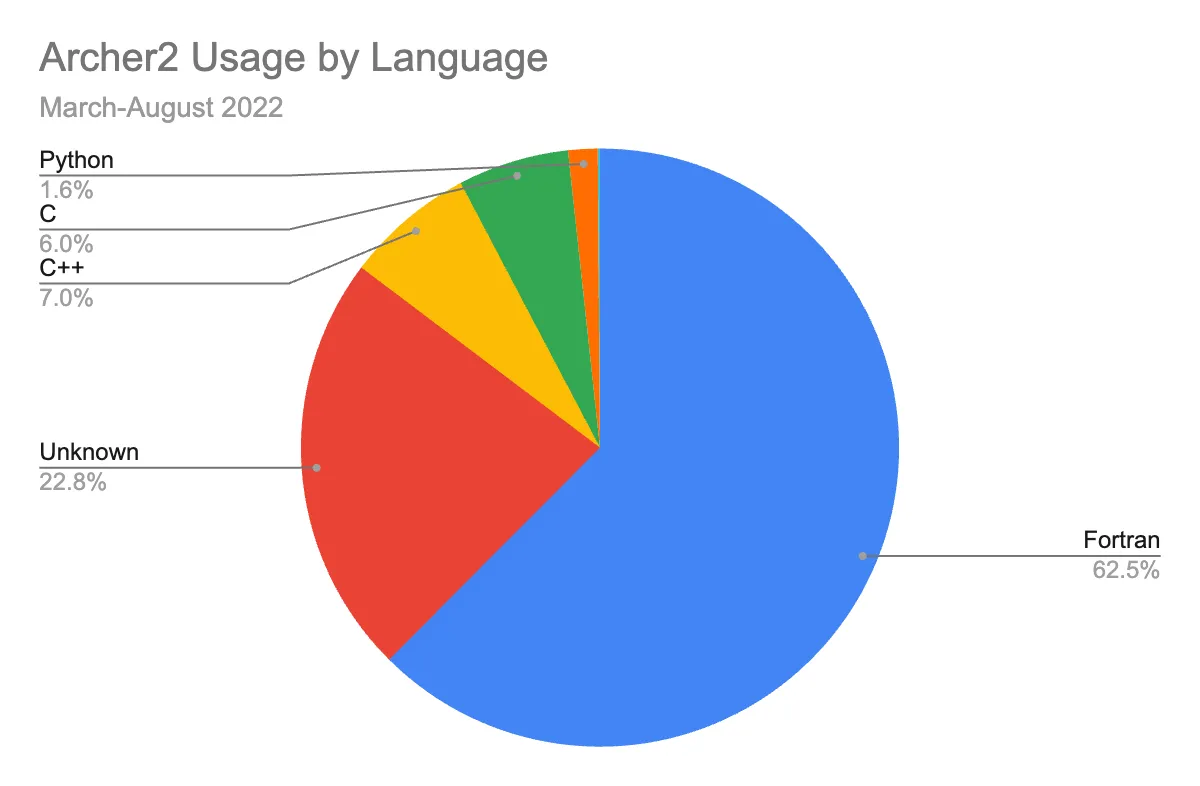
1.3. Resources#
Online dev env
Computer Room
1.4. Useful links#
1.5. Videos recorded from classes#
2024-1s: https://www.youtube.com/playlist?list=PLxbXsIEaf05pxTKg_vBGjzjttosA64dsQ
2023-I: https://www.youtube.com/playlist?list=PLxbXsIEaf05rKMvhEhoRLHL-yJypwsJPK
1.6. Shell/bash#
1.7. git#
1.8. C++#
2. Computer Architecture#
See 01-intro.pdf
Check:
Branch education, computer hardware: https://www.youtube.com/watch?v=d86ws7mQYIg&t=0s
2.1. Review of C++ Programming#
Understand the problem
Edit the code
Compile
Execute and return to the first step
2.1.1. Editing#
Any text editor, but better use an ide like:
Visual studio (local, online)
Vscodium
Emacs
2.1.2. Compiling#
Compilation + Linking
g++ filename.cpp
If the compilation is successful, this produces a file called a.out
on
the current directory. You can execute it as
./a.out
where ./
means “the current directory”. If you want to change the
name of the executable, you can compile as
g++ filename.cpp -o executablename.x
Replace executablename.x
with the name you want for your executable.
Example program
// Mi primer programa
#include <iostream>
int main(void)
{
std::cout << "Hola Mundo!" << std::endl;
return 0;
}
Compilation stages:
Pre-processing: This does not compile. It just executes the precompiler directives. For instance, it replaces the
iostream
header and put it into the source code
g++ -E helloworld.cpp
# This instruction counts how many lines my code has now
g++ -E helloworld.cpp | wc -l
Compilation : Object code
g++ -c helloworld.cpp
Linking and executable: ld, use ldd and nm
g++ helloworld.cpp
2.1.3. Example: Computing the mean of a vector#
#include <iostream>
#include <vector>
#include <cmath>
#include <cstdlib>
#include <algorithm>
#include <numeric>
// function declaration
void fill(std::vector<double> & xdata); // llenar el vector
double average(const std::vector<double> & xdata); // calcular el promedio
int main(int argc, char **argv)
{
// read command line args
int N = std::atoi(argv[1]);
// declare the data struct
std::vector<double> data;
data.resize(N);
// fill the vector
fill(data);
// compute the mean
double result = average(data);
// print the result
std::cout.precision(15);
std::cout.setf(std::ios::scientific);
std::cout << result << "\n";
return 0;
}
// function implementation
void fill(std::vector<double> & xdata)
{
std::iota(xdata.begin(), xdata.end(), 1.0); // 1.0 2.0 3.0
}
double average(const std::vector<double> & xdata)
{
// forma 1
return std::accumulate(xdata.begin(), xdata.end(), 0.0)/xdata.size();
// forma 2
// double suma = 0.0;
// for (auto x : xdata) {
// suma += x;
// }
// return suma/data.size();
}
2.1.4. Loops#
// imprima los numeros del 1 al 10 suando while
#include <iostream>
int main(void)
{
int n;
n = 1;
while (n <= 10) {
std::cout << n << std::endl;
++n;
}
return 0;
}
2.1.5. Condicionales#
// verificar si un numero es par
/*
if (condicion) {
instrucciones
}
else {
instrucciones
}
*/
#include <iostream>
int main(void)
{
int num;
// solicitar el numero
std::cout << "Escriba un numero entero, por favor:" << std::endl;
// leer el numero
std::cin >> num;
// verificar que el numero es par o no
// imprimir
// si el numero es par, imprimir "el numero es par"
// si no, imprimir "el numero es impar"
if (num%2 == 0) {
std::cout << "El numero es par" << std::endl;
}
if (num%2 != 0) {
std::cout << "El numero es impar" << std::endl;
}
//else {
//cout << "El numero es impar" << endl;
//}
return 0;
}